Why is the enrollment GET call in my code below not working? I am receiving a 400 Bad Request error.
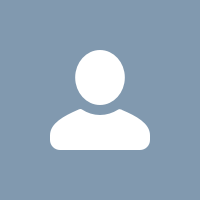
// Define the base URL for the Brightspace API endpoint for enrollments const baseURL = `https://example.com/d2l/api/lp/1.44/enrollments/myenrollments/`; // Define the URL for fetching enrollments with the bookmark parameter let enrollmentsURL = `${baseURL}?bookmark=1`; // Array to store all enrollments let allEnrollments = []; // Fetch all pages of enrollments while (enrollmentsURL) { const enrollmentsResponse = await fetch(enrollmentsURL); const enrollmentsData = await enrollmentsResponse.json(); // Define enrollmentsData here // Add fetched enrollments to the array allEnrollments = allEnrollments.concat(enrollmentsData.Items); // Check if there are more pages if (enrollmentsData.PagingData && enrollmentsData.PagingData.bookmark) { enrollmentsURL = `${baseURL}?bookmark=${enrollmentsData.PagingData.bookmark}`; } else { enrollmentsURL = null; } }
Answers
-
I suspect it's bookmark=1 that is failing as 1 isn't a valid OrgUnitId. Your root site OrgUnitId might work as a suitable default - I'm not sure if 6606 is universal for that.
-
Thanks @Steve.B.446 - You were correct about the bookmark value being the problem, and fixing
the orgunit has resolved the issue. However, I'm still unable to get the expected results.
Here's a quick summary: In our staff training course, a new user can create a sandbox for themselves. I'm using the Whoami, Enrollment, and Create Course APIs. While Whoami and Create Course are working as
expected, the Enrollment API is not. I'm utilising the Enrollment API to search for a sandbox if it's already created and to avoid duplication.
Do you have any advice? Please see the remaining code below.// Check if the course already exists in the user's enrollments const courseExists = allEnrollments.some(enrollment => enrollment && enrollment.CourseName === uniqueName); if (courseExists) { // Display message if the course already exists document.getElementById("message").textContent = `Course "${uniqueName}" already exists in your enrollment.`; return; // Exit function if course already exists }
-
My thoughts would be to use console.log(allEnrollments) before the block of code in your second post to confirm whether the data you are expecting to see is in allEnrollments at the time that you are executing that routine. Are you trying to search allEnrollments before the API calls populating it have finished?
-
Thanks @Steve.B.446 - Yes, using the console log, I can see a list of courses in the browser console with course details. However, the API still creates a course with the same name that already exists in my enrollment. Yes, I am attempting to search all enrollments before a course is created by the API.
Additionally, I'm testing another API in Postman to retrieve all course children in my VLE using the course API. I can retrieve all course children easily, but I'm unable to filter it down by course 'Name' or 'Code'. Instead, I can only filter it down by course Type Id and name. For example, see below:
/d2l/api/lp/{{lpversion}}/orgstructure/6606/children/?ouTypeId=5&Code=Semester
-
The logical question here is whether the course name that is being created as a duplicate exists in the API output you are logging to console. If it's there, the issue is your logic for testing for it existing in that object or the order in which you are doing things. If it's not then the API calls are not producing the output that you expect for some reason.
On your second query, the documentation for that route suggests that OuTypeId is the only valid query parameter. OrgUnitName and OrgUnitCode are only valid parameters for GET /d2l/api/lp/(version)/orgstructure/
-
Hi @Steve.B.446 - I have tried using the code below, and the APIs are working, including the enrollment one. However, the course is still not appearing in my enrollment list. The console only displays 1 page enrollments with 100 results, which suggests that the API is only searching the first page and, since the course is not found there, it creates a new course with the same name. I am unsure how to get the API to search through all my enrollments to find the course. Do you have any suggestions?- thanks
// Function to fetch all enrollments
async function fetchEnrollments() {
const baseURL = `https://www.example.com/d2l/api/lp/1.44/enrollments/myenrollments/`;
let enrollmentsURL = baseURL;
let allEnrollments = [];// Fetch all pages of enrollments
while (enrollmentsURL) {
const enrollmentsResponse = await fetch(enrollmentsURL);
const enrollmentsData = await enrollmentsResponse.json();
allEnrollments = allEnrollments.concat(enrollmentsData.Items);// Check if there are more pages
if (enrollmentsData.PagingData && enrollmentsData.PagingData.HasMoreItems) {
enrollmentsURL = `${baseURL}?bookmark=${enrollmentsData.PagingData.Bookmark}`;
} else {
enrollmentsURL = null;
}
}return allEnrollments;
} -
If you only have 100 items, it sounds like either your search routine is happening after only one page of data has been retrieved or the multiple queries are overwriting the previous one rather than concatenating them.
-
Hi @Steve.B.446 - I've resolved the issue, and now my code is functioning as expected. Instead of using the enrollment API, I switched to the orgstructure API, which works perfectly for my needs.